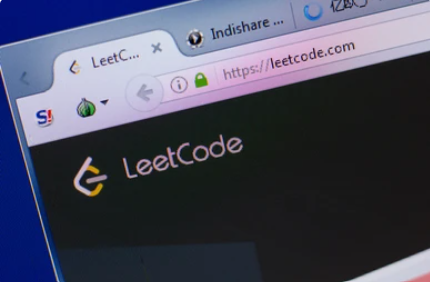
During a technical interviewin the past, I was presented with a challenging leetcode problem: the “Product of Array Except Self.” As I analyzed and solved it under time constraints, I not only completed the task but also deepened my understanding of algorithm design and optimization. The problem, though seemingly straightforward, tested my ability to think critically about time and space efficiency. This blog post outlines my approach to solving it, lessons learned, and why problems like these are relevant to real-world software engineering challenge.
LeetCode’s “Product of Array Except Self” is a classic example of a problem that tests a developer’s ability to think about algorithm optimization, especially when constraints are introduced. This problem has real-life applications and serves as a stepping stone for understanding complex data structures, time complexity optimization, and memory management. Below, we dive deeper into solving the problem efficiently, discuss its real-life significance, and examine its relevance to system design and software engineering practices.
Table of Contents
Problem Breakdown
The problem gives an integer array nums and asks you to return an array answer such that answer[i] is the product of all the elements in the array except nums[i]. The catch is that the solution needs to run in O(n) time and avoid using division, making it a good exercise in optimization.
Example:
Input: nums = [1,2,3,4]
Output: [24,12,8,6]
In the example above, answer[0] = 2*3*4 = 24, answer[1] = 1*3*4 = 12, answer[2] = 1*2*4 = 8, and answer[3] = 1*2*3 = 6.
Approach to Solve the Problem
- Naive Approach with Division: The simplest solution would be to compute the product of the entire array and then divide by each element. While this would give the correct answer, it violates the problem’s constraints, specifically prohibiting the use of division.
- Optimized Approach with Two Arrays: A better approach involves creating two auxiliary arrays:
- Prefix Products Array: This stores the product of all elements before the current index.
- Suffix Products Array: This stores the product of all elements after the current index.
By combining these two arrays, we can compute the answer in O(n) time complexity.
- Final Optimized Solution in O(1) Space: The most space-efficient solution eliminates the need for extra arrays. Instead, we can compute the result in place by:
- Storing the prefix products directly in the answer array.
- Then, using a single variable to store and update the suffix product while iterating through the array from right to left.
This approach optimizes the space complexity to O(1) (excluding the space for the output array), which is often a critical requirement in real-life systems that need to handle large datasets with minimal memory usage.
Code Example in Java:
public int[] productExceptSelf(int[] nums) {
int[] prodArray = new int[nums.length];
int product = 1;
prodArray[0] = nums[0];
for(int i=1; i< nums.length; i++) {
prodArray[i] = prodArray[i-1] * nums[i];
}
for(int i = prodArray.length-1; i >= 0; i--) {
if(i==0) {
prodArray[i] = product;
} else {
prodArray[i] = prodArray[i-1] * product;
product = product * nums[i];
}
}
return prodArray;
}
Explanation:
The first loop computes the prefix product, which is stored in the answer array.
The second loop computes the suffix product using a variable product and multiplies it with the corresponding prefix product already stored in the answer array.
This solution runs in O(n) time and uses O(1) extra space, making it highly efficient.
Full Code is available here in my Github
Real-Life Relevance of LeetCode DSA Problems
LeetCode problems like this one are not only designed to test algorithmic thinking but also have direct applications in software engineering. Let’s explore why mastering these problems is important for real-world systems.
1. Optimizing Performance in Large-Scale Systems
In real-world applications, performance optimization is crucial, especially when working with large datasets. Software engineers often deal with operations that need to process arrays, lists, or matrices of numbers efficiently. Algorithms like the one described above help ensure that even with large-scale data, we can perform computations in linear time (O(n)) and without unnecessary space consumption.
Consider a scenario in a high-frequency trading system where the processing of numerical data (e.g., stock prices, trading volumes) is critical. By understanding and applying optimization techniques such as prefix and suffix product arrays, a software engineer can design systems that process data in a time-efficient manner, reducing latency and improving throughput.
2. Memory Management
Memory management is a key component of system design, particularly in environments with strict memory constraints. In many embedded systems, IoT devices, or mobile applications, memory is a limited resource. The solution above, which uses O(1) space (aside from the output array), ensures that the program does not require additional space proportional to the input size. This principle can be extended to a wide variety of real-world applications where space is a premium resource, such as mobile apps or cloud-based systems with high availability and low latency requirements.
3. Concurrency and Parallel Processing
In some systems, the ability to compute results in parallel can drastically reduce the overall time complexity. While this specific problem may not directly involve parallel processing, the concept of breaking down a problem into smaller subproblems (like calculating prefix and suffix products) is fundamental to parallel computing. In large distributed systems, splitting tasks into smaller parts that can be processed concurrently is essential to scaling applications effectively.
For example, in a distributed database system, data can be split across multiple servers, and computations can be performed in parallel on different segments of the data. LeetCode problems teach the fundamentals of such decomposition, which is applicable in these contexts.
4. System Design Interviews
Many software engineering roles, especially at top tech companies, require candidates to have strong problem-solving skills, which is why LeetCode problems are often included in technical interviews. The ability to quickly understand constraints and come up with efficient solutions is highly valued. Moreover, the process of optimizing space and time complexity is frequently required in system design interviews. Understanding these concepts allows engineers to design scalable, efficient systems from the ground up.
5. Real-World Problem Solving with Algorithms
Real-world systems frequently involve dealing with operations like searching, sorting, merging, and transforming data. For instance, problems related to handling user input, processing large files, or querying databases all require efficient algorithm design to meet performance requirements. The problem-solving techniques learned through LeetCode problems have direct applications in such tasks. Whether it’s dealing with large datasets, handling transactions, or processing complex queries, understanding algorithms helps engineers design solutions that can scale effectively and reliably.
Conclusion
LeetCode problems, such as “Product of Array Except Self,” provide valuable learning experiences that extend far beyond coding practice. By mastering these problems, software engineers gain insights into optimization, memory management, and scalable system design—skills that are crucial in real-world engineering. In addition to improving coding proficiency, these problems help build the mindset needed to approach complex, real-world challenges with efficiency and scalability in mind.
If you’re preparing for interviews or working to enhance your problem-solving skills, understanding and solving these types of problems will make a significant difference in your ability to tackle system design challenges effectively in the software industry.